Last Updated on February 12, 2024
This lab builds on the previous one, so check that one out before reading this one. Here are the instructions:
The previous lab wrote a function to return a dictionary of letter counts. In an earlier chapter, we wrote a turtle program that could draw a histogram.
Combine these two ideas together to create a function that will take a string and create a histogram of the number of times each letter occurs. Make sure it is in alphabetical order from left to right.
Count the number of times each letter occurs. Keep the count in a dictionary.
Get the keys from the dictionary, convert them to a list, and sort them.
Iterate through the keys, in alphabetical order, getting the associated value (count).
Make a histogram bar for each.
Here is the code I started with from the previous lab:
def countAll(text):
wordDict = {}
for char in text:
if char not in wordDict and char.isalpha():
wordDict[char] = text.count(char)
return wordDict
print(countAll("banana"))
print(countAll("curly fries 123!"))
Since I already did the dictionary thing in the previous lab, the first order of business was to convert the dictionary into a sorted list. Here is that code:
def countAll(text):
wordDict = {}
for char in text:
if char not in wordDict and char.isalpha():
wordDict[char] = text.count(char)
return wordDict
def sortList(text):
wordDict = countAll(text)
alphaList = list(wordDict.items())
alphaList.sort()
return alphaList
print(sortList("banana"))
print(sortList("curly fries 123!"))
Result:

The next thing to do was to draw a histogram with the values. Histograms are something I’ve done in several labs and exercises for this book, so I just took the code that I had already previously written and adapted it for this. I also added in a line to convert all letters to lowercase since programming languages tend to treat upper and lower case letters as separate characters.
import turtle
def countAll(text):
wordDict = {}
text = text.lower()
for char in text:
if char not in wordDict and char.isalpha():
wordDict[char] = text.count(char)
return wordDict
def sortList(text):
wordDict = countAll(text)
alphaList = list(wordDict.items())
alphaList.sort()
return alphaList
def writeData(tt, letter, height):
tt.up()
tt.left(90)
tt.forward(height+1)
tt.right(90)
tt.forward(10)
tt.write(letter + ": " + str(height), font=("arial",20))
tt.forward(30)
tt.right(90)
tt.forward(height+1)
tt.left(90)
def drawBar(t, height):
""" Get turtle t to draw one bar, of height. """
t.begin_fill()
t.left(90)
t.forward(height)
t.right(90)
t.forward(40)
t.right(90)
t.forward(height)
t.left(90)
t.end_fill()
def getMaxheight(htList):
maxht = 0
for item in htList:
if item[1] > maxht:
maxht = item[1]
return maxht
xs = sortList(input("What word(s) would you like sorted?"))
maxheight = getMaxheight(xs)
numbars = len(xs)
border = 2
wn = turtle.Screen()
wn.setworldcoordinates(0, 0, 40*numbars+border, maxheight+border)
wn.bgcolor("darkslategrey")
tess = turtle.Turtle()
tess.color("thistle")
tess.fillcolor("maroon")
alex = turtle.Turtle()
alex.color("thistle")
for a in xs:
drawBar(tess, a[1])
writeData(alex, a[0], a[1])
wn.exitonclick()
Result for input “Supercalifragilisticexpialidocious”:
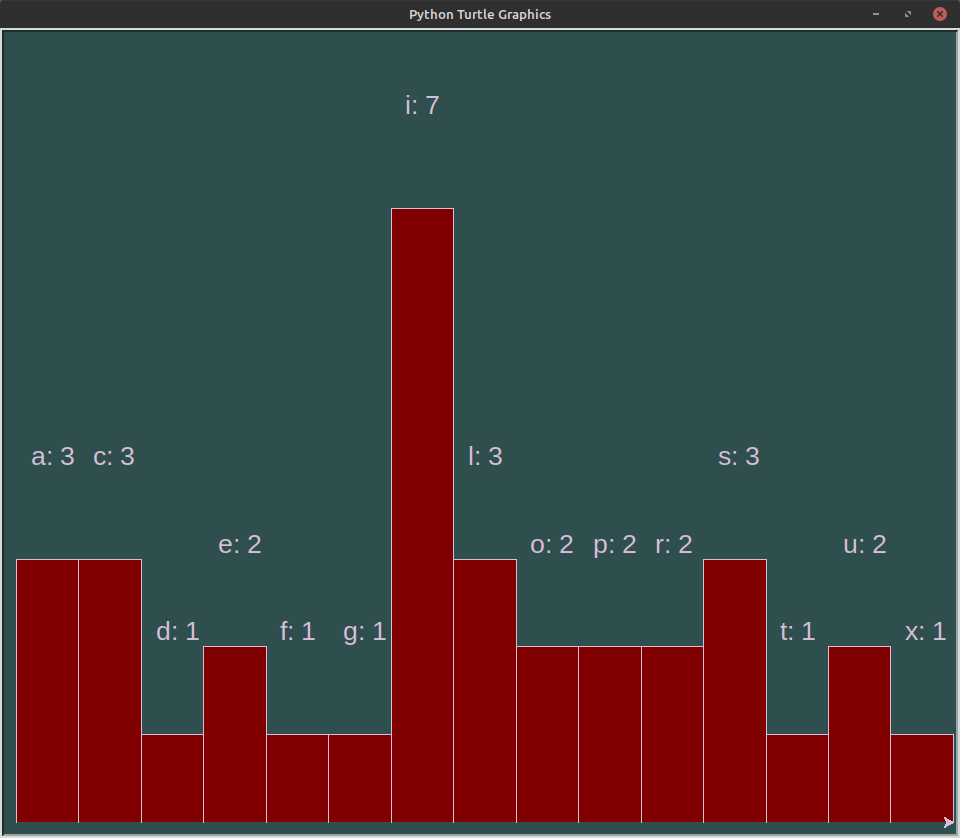